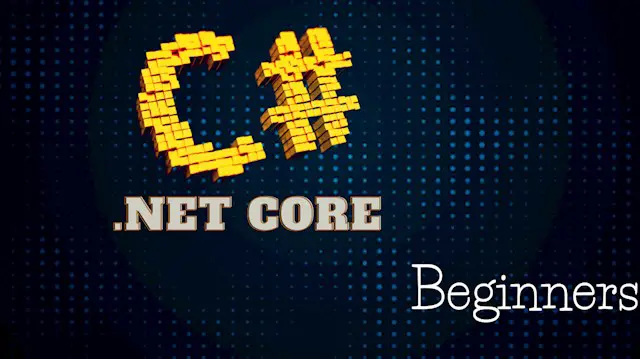
C# Basics with .NET Core for Beginners: Learn by Coding
Master C# Basics with .NET Core - The Most in-depth Course with Live Coding and Practical Assignments in Every Topic
Thomas Plathanath Mathew
Summary
- Reed courses certificate of completion - Free
- Tutor is available to students
Add to basket or enquire
Overview
-
Master the basics of C# and .NET Core
-
Understand fundamentals of computer programming
-
Learn to visually organize the step-by-step process of a program
-
Use primitive data types and expressions
-
Learn to control the flow of program execution using decision making and iteration statements
-
Implement exception handling and data validation
-
Work with single-dimensional and multi-dimensional arrays
-
Learn effective string manipulations and work with date and time
-
Learn the difference between value types and reference types
-
Learn and implement object-oriented programming concepts
-
Work with structures
-
Learn enum, and collections(ArrayList, HashTable, and List<T>) and implement in real-life examples
-
Manipulate text and binary files
-
Understand nullable and random class
-
Learn how to debug C# applications effectively
Curriculum
-
Introduction 04:24
-
Computer Programming Basics 55:04
-
Introduction to .NET Core And C# 43:34
-
Variables, Primitive Data Types, and Constants 59:42
-
Basic Output Statements 1:11:56
-
Basic Input Statements 1:10:25
-
Operators and Expressions 1:07:34
-
Decision Making Statements 1:22:04
-
Iteration Statements 1:27:12
-
Single Dimensional And Multi Dimensional Arrays 1:29:44
-
Exception And Exception Handling 57:47
-
Validating Input Data 1:05:37
-
String Manipulation 1:34:22
-
Methods 51:23
-
Object Oriented Programming 2:57:31
-
Value and Reference Types 19:18
-
Structures 28:29
-
enum and Collections 2:40:13
-
File Handling 2:38:35
-
Scope of Variables, Nullables and Random Class 35:45
-
Date and Time Manipulation 1:08:36
-
Debugging Tools in Visual Studio 21:03
-
Final Project - Inventory Management 2:05:59
-
What's Next? 03:24
Course media
Description
C# was named one of the most popular programming languages for server-side programming, app development, web development, and game development.
Why Learn C#?
C# is a versatile and modern programming language created by Microsoft. It is evolving and widely used by developers to build a variety of applications including desktop applications, web applications, native Android and iOS mobile apps, web services and web API, Azure cloud applications and services, and game development.
.NET Core is an open-source, free, multi-platform framework from Microsoft and it replaces .NET Framework. .NET Core 3.0 adds support for C# 8.0 and can be used to develop cross-platform applications.
Mastering C# programming with .NET Core lays the foundation for many different career paths with prospective options. You can mold your career as an ASP.NET web developer, desktop developer, or mobile application developer. You can enter the world of game development too. Thorough knowledge of the fundamentals helps you to switch to a different technology stack.
If you see any of the above is the right path for your career, then this course is exactly where you need to start.
Why this course?
This course is the best and most in-depth course in C# basics with .NET Core from Instructor Thomas Mathew. As a Professor, Thomas has already taught over 20,000+ students to code in in-person classes. Thomas teaches you the basics of C# programming in a structured, simple, and tail-to-head manner. This course is presented in such a way that even a novice learner can understand the topics. Every section comes with a collection of lectures on related topics and a quiz. Each programming concept is illustrated with a live coding demo application.
At the end of the course, you will develop a project utilizing all the skills that you learn throughout this course. This course reforms you from a beginner to a pro.
‘Explore Coding…Discover Career’
Who is this course for?
- Beginners: This course is suitable for anyone who wants to learn to code, and no previous experience is required. The course starts from the scratch
- Intermediates: Refresh your C# knowledge in .NET Core
- Anyone who want to take their coding skills to the next level by mastering the fundamentals of Object-Oriented Programming
Requirements
-
Visual Studio Community Edition IDE (Free), and the course includes a demo on how to download and install it
Career path
.NET Developer
Full Stack C#.Net Developer
C# / .NET Software Developer
Web Developer
Questions and answers
Is there any time limit to finish C# language course?
Answer:Hi Sajid, Hope you are doing good. Happy to know that you are prepared to start your coding career in C# with .Net Core. There is no time limit to finish this course. Once enrolled, this course will be available to you for lifetime. FYI, I am in the process of publishing the next level of this course as well. All the best for your journey with C#. Thanks & Regards, Prof. Thomas Mathew
This was helpful.
Certificates
Reed courses certificate of completion
Digital certificate - Included
Will be downloadable when all lectures have been completed
Reviews
Legal information
This course is advertised on reed.co.uk by the Course Provider, whose terms and conditions apply. Purchases are made directly from the Course Provider, and as such, content and materials are supplied by the Course Provider directly. Reed is acting as agent and not reseller in relation to this course. Reed's only responsibility is to facilitate your payment for the course. It is your responsibility to review and agree to the Course Provider's terms and conditions and satisfy yourself as to the suitability of the course you intend to purchase. Reed will not have any responsibility for the content of the course and/or associated materials.